// ==UserScript==
// @name 智能彩虹文本转换器Pro
// @namespace http://tampermonkey.net/
// @version 0.6
// @description 实时中文彩虹色转换工具,带右下角浮动控制面板
// @author Panndora
// @match *://bbs.oldmantvg.net/*
// @grant none
// ==/UserScript==
(function() {
'use strict';
// HSL转RGB函数
function hslToRgb(h, s, l) {
s /= 100;
l /= 100;
const k = n => (n + h/30) % 12;
const a = s * Math.min(l, 1 - l);
const f = n => l - a * Math.max(-1, Math.min(k(n)-3, 9-k(n), 1));
return {
r: Math.round(255 * f(0)),
g: Math.round(255 * f(8)),
b: Math.round(255 * f(4))
};
}
// RGB转HEX函数
function rgbToHex(r, g, b) {
return "#" + [r,g,b].map(x => {
const hex = x.toString(16);
return hex.length === 1 ? "0" + hex : hex;
}).join("");
}
// 生成彩虹色系(36色深色系)
function generateRainbowColors() {
const colorSegments = [
{ start: 0, end: 30, count: 3 }, // 红→橙
{ start: 30, end: 60, count: 3 }, // 橙→黄
{ start: 60, end: 120, count: 6 }, // 黄→绿
{ start: 120, end: 240, count: 12 },// 绿→蓝
{ start: 240, end: 270, count: 3 }, // 蓝→靛
{ start: 270, end: 300, count: 3 }, // 靛→紫
{ start: 300, end: 360, count: 6 } // 紫→红
];
const colors = [];
colorSegments.forEach(segment => {
const {start, end, count} = segment;
const step = (end - start)/(count - 1);
for(let i = 0; i < count; i++) {
let hue = start + step * i;
hue = hue >= 360 ? hue - 360 : hue;
const rgb = hslToRgb(hue, 100, 40);
colors.push(rgbToHex(rgb.r, rgb.g, rgb.b));
}
});
return colors;
}
const rainbowColors = generateRainbowColors();
// 创建样式
const style = document.createElement('style');
style.textContent = `
#colorConverter {
position: fixed;
bottom: 80px;
right: 20px;
background: white;
border: 1px solid #ccc;
padding: 20px;
z-index: 10000;
width: 250px;
font-family: 'Microsoft YaHei';
box-shadow: 0 2px 10px rgba(0,0,0,0.1);
display: none;
max-height: 80vh;
overflow-y: auto;
}
#triggerBtn {
border: 0;
background-color: #090;
color: #fff;
cursor: pointer;
height: 38px;
padding: 5px;
float: right;
margin-right: 10px;
text-align: right;
display: inline-block;
}
#inputText {
width: 100%;
height: 100px;
margin: 10px 0;
resize: vertical;
}
#copyButton {
padding: 8px 16px;
background: #2196F3;
color: white;
border: none;
cursor: pointer;
margin-top: 10px;
}
#outputPreview {
width: 100%;
min-height: 150px;
border: 1px solid #eee;
padding: 10px;
margin-top: 10px;
white-space: pre-wrap;
word-wrap: break-word;
overflow-y: auto;
max-height: 300px;
}
.button-group { margin: 10px 0; }
`;
document.head.appendChild(style);
// 创建触发按钮
const triggerBtn = document.createElement('button');
triggerBtn.id = 'triggerBtn';
triggerBtn.textContent = '彩虹字体';
//document.body.appendChild(triggerBtn);
const targetContainer = document.querySelector('.Tp1');
if (targetContainer) {
targetContainer.insertBefore(triggerBtn, targetContainer.firstChild);
triggerBtn.style.marginRight = '10px'; // 与提交按钮保持间距
}
// 创建转换窗口
const converter = document.createElement('div');
converter.id = 'colorConverter';
converter.innerHTML = `
<h4 style="margin:0 0 15px">老男人彩虹字转换器
<button id="closeBtn" style="float:right; background:none; border:none; cursor:pointer; font-size:1.2em">×</button>
</h4>
<textarea id="inputText" placeholder="输入中文/英文/符号,实时预览彩虹效果..."></textarea>
<div id="outputPreview"></div>
<button id="copyButton">复制转换后的彩虹字</button>
`;
document.body.appendChild(converter);
// 实时转换函数
function convertText() {
const input = document.getElementById('inputText').value;
let html = '';
const colorCount = rainbowColors.length;
let charCount = 0;
for (const char of input) {
const cycle = Math.floor(charCount / colorCount);
const index = charCount % colorCount;
const colorIndex = (cycle % 2 === 0) ? index : (colorCount - 1 - index);
html += `<span style="color:${rainbowColors[colorIndex]}">${char}</span>`;
charCount++;
}
document.getElementById('outputPreview').innerHTML = html;
}
// 复制到剪贴板
// 复制到剪贴板(核心修改部分)
async function copyToClipboard() {
const output = document.getElementById('outputPreview');
const htmlContent = output.innerHTML;
try {
// 创建包含HTML内容的Blob
const blob = new Blob([htmlContent], { type: 'text/html' });
const clipboardItem = new ClipboardItem({
'text/html': blob,
'text/plain': new Blob([output.textContent], { type: 'text/plain' })
});
// 写入剪贴板
await navigator.clipboard.write([clipboardItem]);
改我('彩虹字已成功复制到剪贴板!');
} catch (err) {
// 回退方案:纯文本复制
try {
await navigator.clipboard.writeText(htmlContent);
改我('已复制纯文本格式的HTML代码(部分应用可能不支持富文本粘贴)');
} catch (err) {
改我('复制失败,请手动选择内容后复制');
output.focus();
}
}
}
// 显示/隐藏逻辑
document.getElementById('triggerBtn').addEventListener('click', () => {
converter.style.display = 'block';
triggerBtn.style.display = 'none';
});
document.getElementById('closeBtn').addEventListener('click', () => {
converter.style.display = 'none';
triggerBtn.style.display = 'block';
});
// 绑定实时转换
document.getElementById('inputText').addEventListener('input', convertText);
// 绑定复制事件
document.getElementById('copyButton').addEventListener('click', copyToClipboard);
})();
我给使用油猴子脚本的伙计们,分享一个我刚刚写的小工具.昨天受到一个论坛里面回帖的影响,我发觉自己手动的改文字的颜色太麻烦了,而且原来的颜值色设置只有那几个颜色要扩展一下,我就写了一个猴子脚本,跑了一下,感觉还不错.好吧,我承认不是我写的,我只是给 AI 提了要求而已.为什么我会把直接把脚本代码贴到论坛里面,而不是发布在有猴子脚本的分享网站上?因为这个脚本就在本网站用没必要发出去,让其他人知道,而且那个分享脚本中文用户最多的网站被Q了祝大家玩的愉快对了,有一个最关键的问题,我要讲一下,好像只可以在高级编辑里面才能够发这种有文字的文本,因为转换出来的是 html 文本.
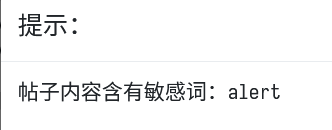
用之前改一下这个地方.
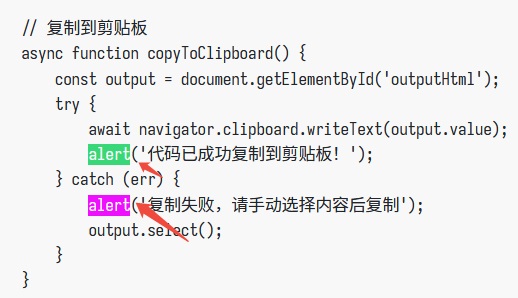